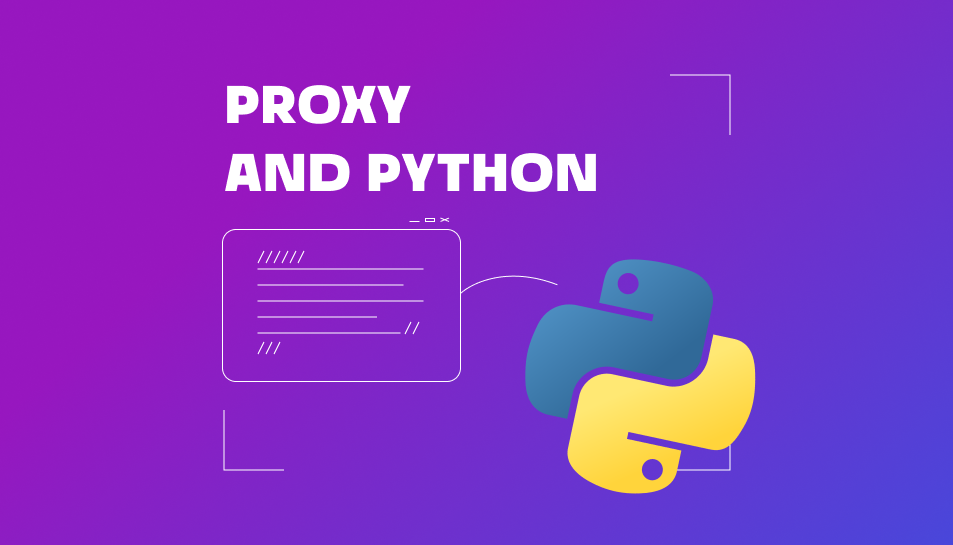
The most common way to send HTTP requests in Python is using the Requests module. It's one of the simplest libraries to use, and when compared to other Python options, building code to extract data from it frequently takes less time.
The best proxies are necessary for web scraping, as experienced users know. Modern websites secure themselves from automation using sophisticated anti-bot technologies. So installing a proxy server is part of creating and maintaining your own scraper in order to get around IP address prohibitions or other web scraping roadblocks.
How to Utilize a Proxy Server with Python Requests
You will need the following requirements before you begin:
- Python 3. Installing the most recent Python version is required.
- Requests. By launching pip install requests, you may add it.
- Coding editor. Make use of whichever editor you choose.
How to Set Up Proxies with Requests
Step 1. Perform the initialization command to configure Python Requests proxy.
import requests |
Step 2. Add your proxy information to the proxies parameter after that.
HTTP proxy:
proxies = { 'http': 'http://host:PORT', 'https': 'http://host:PORT', } |
SOCKS5 proxy:
proxies = { 'http': 'socks5://host:PORT', 'https': 'socks5://host:PORT', } |
Step 3. Let's make a response variable now and feed the proxies parameter to it.
response = requests.get('URL', proxies = proxies) |
Note: Any request method, such as get (), post(), or put(), may be used.
How to Authenticate a Proxy
The password and login information must be sent with the proxy settings in order to authenticate your proxy.
proxies = { 'http': 'http://user:password@host:PORT', 'https': 'http://user:password@host:PORT', } response = requests.get('URL', proxies = proxies) |
How to Set Up Proxy Sessions
You need to start a session and include your proxy if you wish to send out many requests using the same proxy setup. You may achieve this by submitting a request through your proxy settings while supplying a session object.
session = requests.Session() session.proxies = proxies response = session.get('URL') |
How to Set Up Environment Variables
Setting environment variables is necessary if you wish to keep your proxy settings for later usage. In this manner, switching between various proxy settings is simple and requires no coding changes.
Step 1. Your operating system may allow you to export or configure environment variables to the proxy address and port.
For Windows users:
set http_proxy=http://username:password@:PORT set https_proxy=http://username:password@:PORT |
For Linux users:
export http_proxy=http://username:password@:PORT export https_proxy=http://username:password@:PORT |
Step 2. Import the OS library next, after which you should configure proxies dictionary to utilize the environment variables.
import os proxies = { http: os.environ['http_proxy'], https: os.environ['https_proxy'] } requests.get('URL',proxies = proxies) |
How to Rotate Proxies with Requests
You must change your proxies frequently to avoid being banned or having your rate reduced by websites. In any other case, your target will become aware of the excessive number of connection requests coming from one IP.
You'll need a pool of IP addresses to start rotating your proxies. The use of commercial proxy services is strongly advised even though you can obtain free listings. Free IPs can readily expose your data, are unreliable, and may inject advertisements. Contrarily, paid proxies maintain their infrastructure, reducing the likelihood that you will be banned.
Step 1: Import the libraries listed below.
import requests import random |
Step 2: Next, create a list of IP addresses you intend to utilize.
proxy_pool = ['user:password@host:3001', 'user:password@host:3002', 'user:password@host:3003'] |
Step 3: Let's now examine all 10 requests.
for i in range(10): |
1) Choose at random one of your pool of proxies.
proxy = {'http': random.choice(proxy_pool)} |
2) Employ the same proxy to send the request.
response = requests.get('URL', proxies=proxy) |
3) Print the answer.
print(response.text) |
This is the whole script:
import requests import random # Define your proxies proxy_pool = ['user:password@host:3001', 'user:password@host:3002', 'user:password@host:3003'] # Going through 10 requests for i in range(10): # Select a random proxy from the pool proxy = {'http': random.choice(proxy_pool)} # Send the request using the same proxy response = requests.get('URL', proxies = proxy) # Print the response print(response.text) |
Why Use a Proxy with Python Requests?
There are various benefits to processing a HTTP request with the Python Requests package while using a proxy. You may route your requests through several IP addresses and regions by using proxies, which operate as middlemen between your device and the destination server. In situations where you may need to anonymize your queries, access geographically restricted information, or get around rate constraints, this functionality is very helpful for operations like web scraping, data collecting, and security testing. You may improve the adaptability, privacy, and security of your HTTP interactions by establishing proxies with Python Requests, which expands the potential of your applications.
Conclusion
In summary, using proxies into your Python Requests process opens up a world of opportunities and enables you to traverse the digital realm with improved privacy, security, and adaptability. You now have the knowledge and practical skills necessary to incorporate proxies into your projects without difficulty thanks to the exploration of the key components of utilizing proxies with Python Requests that we covered in this definitive guide. Thank you for reading!